Greetings to everyone! Today I bring you a quick introduction to Godot version 4 multiplayer.

If you want a better understanding, I recommend you read the documentation or at least know the differences between server-side and client-side. With that said, let’s go straight into it.
Setup the Network
This is similar to Godot 3.5, but the syntax is a little bit different:
For example, now everything about multiplayer is actually under a global class named “multiplayer” (MultiplayerAPI), meaning when we connect signals, it’s under the multiplayer class.
# GODOT 3.5
get_tree().connect("connected_to_server", self, "_connected_to_server")
# GODOT 4
multiplayer.connected_to_server.connect(self._connected_to_server)
For quick setup, you can use this code:
This code you will add as auto-load and then a create node with lobby and connect the signals, then button to switch scenes. To better understand the code, you can check my previous guide to multiplayer 3.5.
Multiplayer Synchronizer
In this version of Godot, they added the multiplayer synchronizer, which simplifies the programming of multiplayer. Now you need to add it to the player tree, and then just set the properties that you want to synchronize.
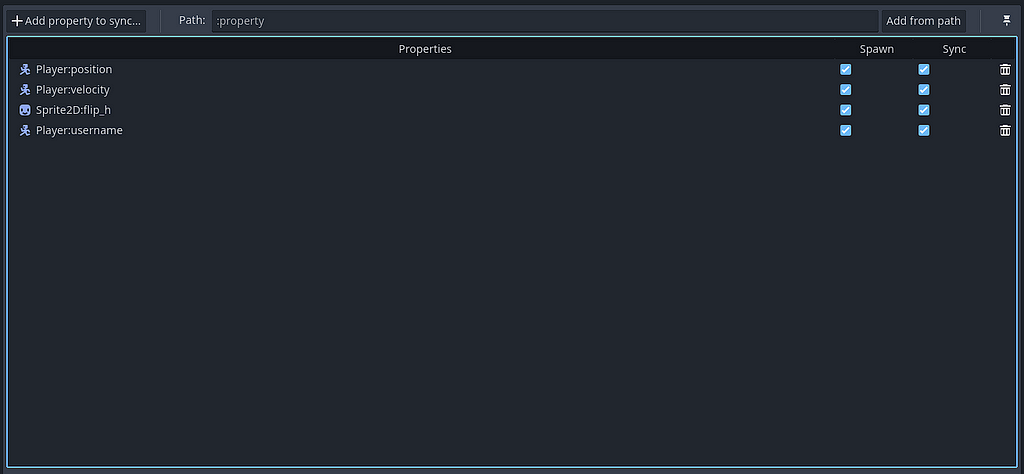
This is so helpful and can save you a lot of time.
Multiplayer Spawner
Another new feature that also simplifies the process of spawning and managing networked objects in multiplayer games
But when I was using it, I fell into lots of errors, and then I just implemented my own spawner. If you want to play with it on your own, I recommend you look up the documentation.
RPC Calls
RPCs are essential for implementing networked gameplay and interactions, and that’s why it’s useful to know how to use them properly.
RPC changed syntax to a much simpler version.
For example, now you don’t have any master, puppet, remote, etc., but you have much simpler syntax.
Syntax of RPC Calls in Godot 4:
You will start with “@”, and then you will specify RPC’s mode, sync, transfer_mode.
# mode, sync, transfer_mode (order doesn't matter)
# reliable -> tcp, unreliable -> udp (spamming), unreliable_ordered
@rpc("any_peer", "call_local", "reliable")
func totaly_sync():
print("Running locally and any_connected_client running this function")
@rpc("call_local")
func local_call():
print("calling just local function")
# You also can use different channels used mostly for optimizations
@rpc(any_peer, 1)
func my_chat_func(message):
print("RPC received on channel 1, message: " + str(message))
# Calling rpc function:
# rpc() == self.rpc
rpc("totaly_sync")
# with arguments:
rpc("my_chat_func", "Hello")
But be careful, you can’t send any node because that could lead to an RCE vulnerability, so you need to work around it. Usually, I declare a list of items that I want to sync and then just send the index through the network.
@onready var items = [
preload("res://path/to/scene.tscn"),
preload("res://path/to/scene.tscn"),
]
@rpc("any_peer", "call_local", "reliable")
func sync_items(index: int) -> void:
spawn_item(items[index])
Controlling the execution flow
The last thing on our list is how to actually control the execution flow. The main way to control the flow is by:
# check whoever executing the code if its the owner of current node
mutiplayer.is_mutliplayer_authority()
# check if its the server
multiplayer.is_server()
# set the ownership of the node
NODE.set_multiplayer_authority()
When you instancing the player, you need to set him as the multiplayer_authority of its node. This will make your life much simpler.
And keep in mind that when using the “_ready()” function, the execution of this function usually happens before the multiplayer_authority is set.
Conclusion
With this article, you should be able to program multiplayer into your game. Sometimes multiplayer can be a pain because something is not syncing properly, and debugging the error is a little bit difficult, but with a cool head, you eventually find the error and gain a little bit more knowledge.
If you enjoyed this article, clap and follow me! Thanks for reading, and I wish you the best luck on your journey.